How to Read the Functions Documentation
This page explains how to use functions described on the Functions page.
The role of JavaScript in the API documentation
The Pathfinder API can be accessed using any general-purpose programming language. This documentation illustrates the use of JavaScript/jQuery to send API requests, but if you prefer to use another language, such as Python or C#, you may.
JavaScript functions
The convenience function OnGetJson()
Some logic is common to all Pathfinder API requests, so you will probably want to use a function to issue the API requests and process any error messages. That is the approach we have taken in this documentation: the function OnGetJson()
below sends a request to our API server. All of our code examples use OnGetJson()
to access the API. If you happen to be using JavaScript/jQuery, you are welcome to copy OnGetJson()
; otherwise, we encourage you to write and use a similar function to issue API requests in a standardised way.
OnGetJson()
implementation:
function OnGetJson(url, data) {
$("#resultjson").show().html("Loading...");
$("#resulttable, #resultchart").hide();
$("#apiresult").html("");
$.support.cors = true;
$.ajax({
url: baseUrl + url + '?id=' + _uuid,
type: 'POST',
contentType: 'application/json',
data: JSON.stringify(data),
dataType: 'json',
success: SuccessHandler,
error: ErrorHandler
});
}
function SuccessHandler(result) {
$("#resultjson").html(JSON.stringify(result, null, 2).replaceAll("\\\\", "\\"));
$("#apiresult").html("Success");
}
function ErrorHandler(xhr, exception) {
var msg = '';
if (xhr.status === 0) {
msg = 'Not connect.\n Verify Network.';
} else if (xhr.status == 401) {
msg = JSON.parse(xhr.responseText);
} else if (xhr.status == 404) {
msg = 'Requested page not found. [404]';
} else if (xhr.status == 500) {
msg = 'Internal Server Error [500].';
} else if (exception === 'parsererror') {
msg = 'Requested JSON parse failed.';
} else if (exception === 'timeout') {
msg = 'Time out error.';
} else if (exception === 'abort') {
msg = 'Ajax request aborted.';
} else {
msg = 'Uncaught Error.\n' + xhr.responseText;
}
$('#resultjson').html(msg);
$("#apiresult").html("Failed");
}
JavaScript wrapper functions
The API consists of a number of API functions. The API Test View demonstrates how each can be used, and the code examples appearing in this documentation are drawn from there. In the API Test View, each API function is triggered by a button-click, so for each API function, you will see a JavaScript wrapper function, acting as an event handler, which calls the above convenience function OnGetJson()
with a URL specifying which API function is to be used. Thus each code example has this structure:
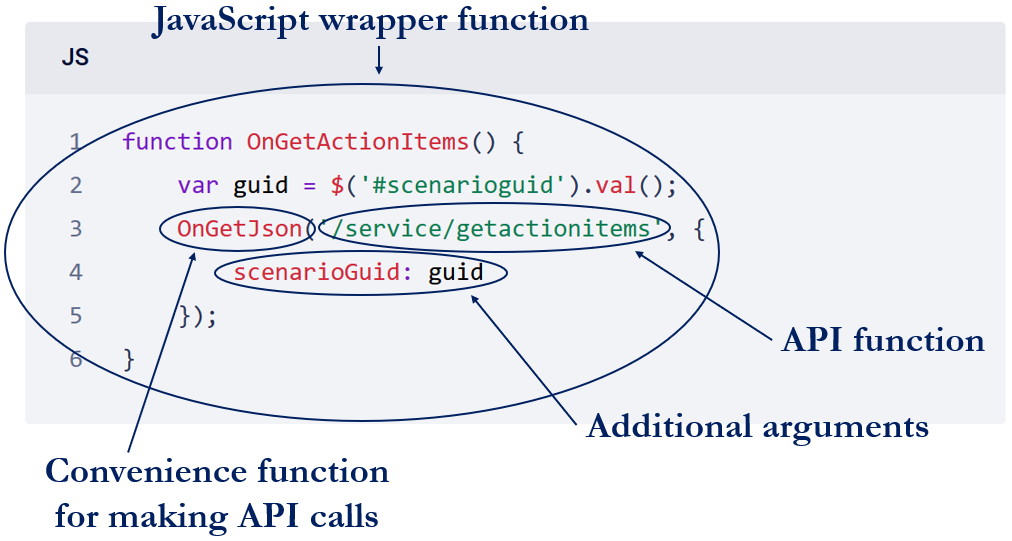